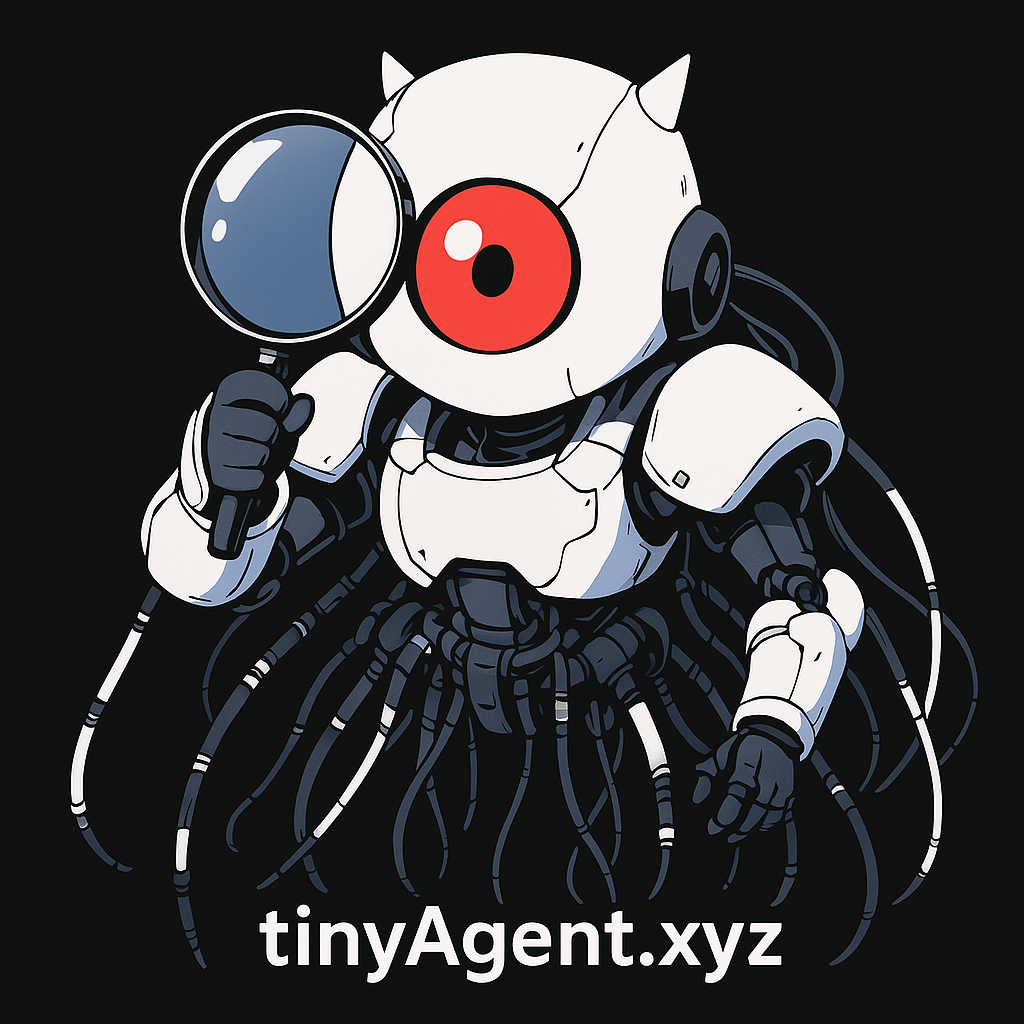
Turn Functions into AI Tools
tinyAgent lets you wrap any Python function with a simple decorator, combine agents to solve complex problems, and leverage experimental meta-agents.
from tinyagent.decorators import tool
from tinyagent.agent import tiny_agent
@tool
def calculate_sum(a: int, b: int) -> int:
"""Return the sum of two integers."""
return a + b
if __name__ == "__main__":
agent = tiny_agent(tools=[calculate_sum])
query = "calculate the sum of 5 and 3"
result = agent.run(query, expected_type=int)
print(f"Query: '{query}' -> Result: {result}")
FUNCTIONS AS AGENTS
def hello()
@tool
"Hello!"